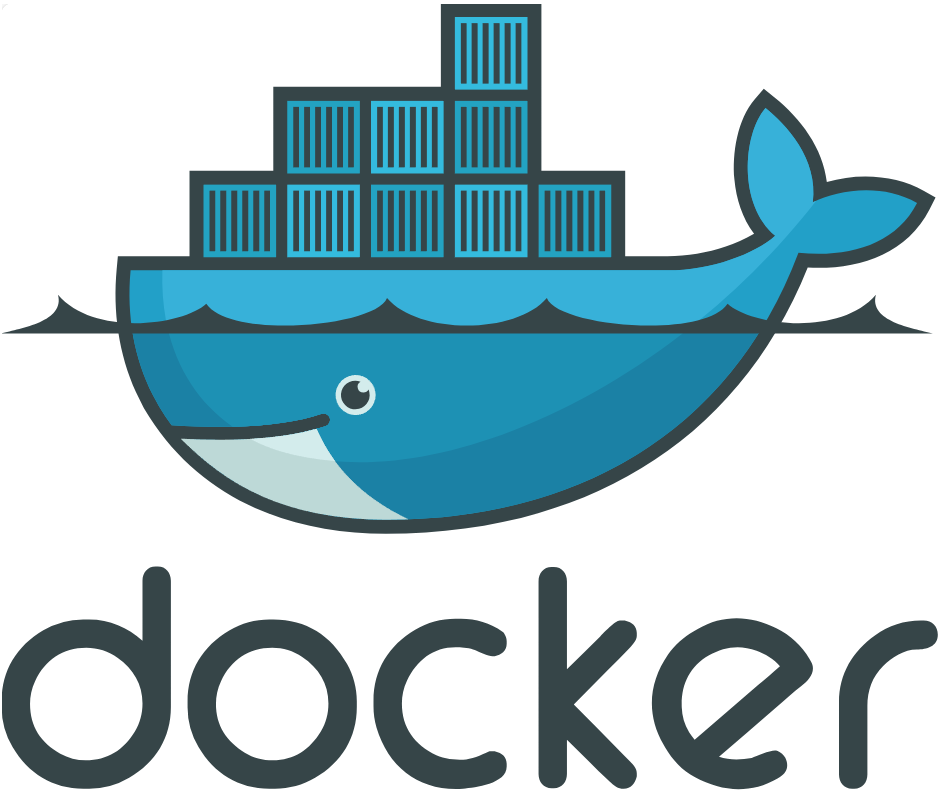
What is docker #
Docker is an open platform for developing, shipping, and running applications. Docker enables you to separate your applications from your infrastructure so you can deliver software quickly. With Docker, you can manage your infrastructure in the same ways you manage your applications. By taking advantage of Docker’s methodologies for shipping, testing, and deploying code, you can significantly reduce the delay between writing code and running it in production.
This small overview is my first experience with docker on the commandline, i did a bit of research online read some forums and did hello world examples.
Installation #
sudo apt update
sudo apt install docker-ce docker-ce-cli containerd.io docker-buildx-plugin docker-compose-plugin
Hello world #
install with:
docker pull hello-world
run with:
docker run hello-world
output:
Hello from Docker!
This message shows that your installation appears to be working correctly.
To generate this message, Docker took the following steps:
1. The Docker client contacted the Docker daemon.
2. The Docker daemon pulled the "hello-world" image from the Docker Hub.
(amd64)
3. The Docker daemon created a new container from that image which runs the
executable that produces the output you are currently reading.
4. The Docker daemon streamed that output to the Docker client, which sent it
to your terminal.
To try something more ambitious, you can run an Ubuntu container with:
$ docker run -it ubuntu bash
Share images, automate workflows, and more with a free Docker ID:
https://hub.docker.com/
For more examples and ideas, visit:
https://docs.docker.com/get-started/
View images #
command:
sudo docker images
output:
REPOSITORY TAG IMAGE ID CREATED SIZE
hello-world latest 7884a9d2ecf1 19 months ago 4.85kB
Apache httpd webserver #
Installation #
Install:
sudo docker pull httpd
run image with port mapping like:
sudo docker run -p <host_port>:<container_port> <image_name>
running httpd in foreground/console:
sudo docker run -p 8080:80 httpd
you will see a container ID number, to stop the container use the command:
sudo docker stop 4f2b79f3ab465816942b13a89a2543fffbadd424d02716e56bed013875f5b4fa
Container name #
So you can set a NAME for the container, wich is more maintainanable then to track ID numbers:
sudo docker run -d -p 8080:80 --name MYSERVER httpd
Now you can stop the container easily with:
sudo docker stop MYSERVER
List running containers #
To list running containers, use the command:
sudo docker container ls
Output:
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
eed1b6222636 httpd "httpd-foreground" 6 seconds ago Up 4 seconds 0.0.0.0:8080->80/tcp, [::]:8080->80/tcp MYSERVER
To start the container again you can use:
sudo docker start MYSERVER
Edit http files #
To edit index.html file (wich contains “It Works!” you first have to enter (go inside) the container:
sudo docker exec -it SOMEN bash
The container is pretty empty and contains only the basic programs, you need to install a text editor first for example “nano”:
apt-get update && apt-get install -y nano
Now you can edit the index.html file and exit the container:
sudo nano /usr/local/apache2/htdocs/index.html
exit
Copy files directly to a container #
For example copy a index.html file inside a container file:
sudo docker cp /home/pi/index.html MYSERVER:/usr/local/apache2/htdocs/index.html
Mount docker folder to local folder #
for example create a folder in your home folder:
sudo mkdir htdocs
Then run the container as follows:
sudo docker run -d --name MYSERVER -p 8083:80 --mount type=bind,source="$(pwd)/htdocs",target=/usr/local/apache2/htdocs/ httpd
Now you can place and edit files locally that a re being run by the container
Stop all containers #
A command to stop all containers:
sudo docker container kill $(docker container ls -q)
Sudo errors, add docker to sudo #
Use these command if you get sudo erros and to add docker to the sudo group
sudo groupadd docker
sudo usermod -aG docker ${USER}
sudo chmod 666 /var/run/docker.sock
Purge all containers #
Purging All Unused or Dangling Images, Containers, Volumes, and Networks
Docker provides a single command that will clean up any resources — images, containers, volumes, and networks — that are dangling (not tagged or associated with a container):
docker system prune
To additionally remove any stopped containers and all unused images (not just dangling images), add the -a flag to the command:
docker system prune -a
PHP #
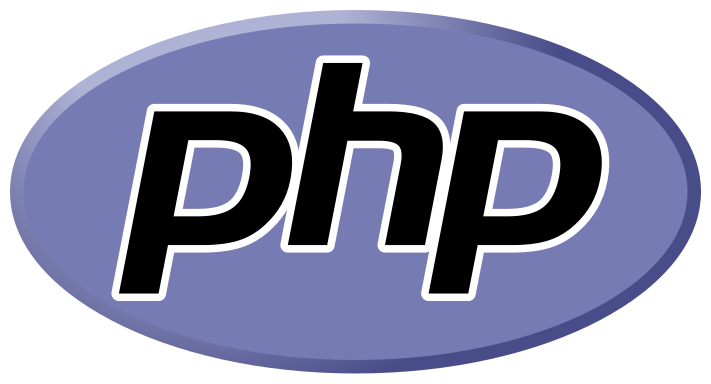
To use php with apache (httpd) you need the following container:
sudo docker pull php:apache
Now you can run the container for example:
sudo docker run -d --name MYSERVER -p 8080:80 --mount type=bind,source="$(pwd)/htdocs",target=/var/www/html/ php:apache
To test things out you can place a index.php file inside you local folder for example: /home/pi/htdocs/index.php with the contents:
<? phpinfo(); ?>
and then go to your [ip]:8080 address to view the php info page.