
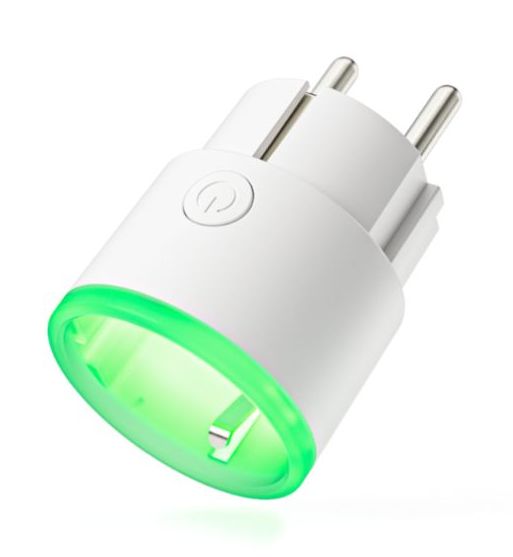
Micropython Raspberry Pico – example #
Library requests_1.py #
The standard micropython build-in request library could give unexpected timeouts on GET command, even returned data is not stored properly, so a pautch has been made.
Source: https://github.com/shariltumin/bit-and-pieces/tree/main/web-client
This is a copy of the urequests library with a very slight modification to the content()
method, using socket.recv(blk)
instead of socket.read()
.
The urequests/requests is a frozen module in the standard MicroPython firmware. The modified version is named “requests_1.py” so as not to conflict with the standard version. To make use of this modified version:
- copy requests_1.py to your flash
- import requests_1 as request
- req = requests.get(URL, timeout=10)
This is not an official version of the library and it is probably not good practice to use a modified version of the standard library. Please use the modified version of the library as a learning tool. If you find that this solves your problem, please request a modification from the MicroPython development team.
Code to scan for Homewizard devices:
import network
import requests_1 as requests
import time
import json
ssid = "Matrix"
password = "139726845$e"
def connect_wifi():
wlan = network.WLAN(network.STA_IF)
wlan.active(True)
wlan.connect(ssid, password)
print("Verbinding maken met WiFi...")
while not wlan.isconnected():
time.sleep(1)
ip = wlan.ifconfig()[0]
print("Verbonden! IP:", ip)
# Subnet bepalen: pak de eerste drie octetten
subnet = ".".join(ip.split(".")[:3]) + "."
print("Subnet:", subnet)
return subnet # Geeft het subnet terug
# Netwerkscan naar HomeWizard plug
def scan_network(subnet):
api_endpoint = "/api"
api_data_endpoint = "/api/v1/data"
plugs = [] # Lijst om gevonden HomeWizard plugs op te slaan
for i in range(5, 20): # Scan bijvoorbeeld van .5 tot .20
target_ip = subnet + str(i)
url = f"http://{target_ip}{api_endpoint}"
print(f"Scant: {target_ip}...")
try:
response = requests.get(url, timeout=1)
info = response.json()
if info.get('product_type') == "HWE-SKT": # Plug is een HomeWizard SOCKET
url = f"http://{target_ip}{api_data_endpoint}"
response = requests.get(url, timeout=1)
data = response.json()
if data.get('wifi_ssid'): # Plug is actief
print(f"HomeWizard PLUG gevonden op {target_ip} - Serial: {info['serial']} - WiFi strength: {data['wifi_strength']}")
plugs.append({
"ip": target_ip,
"serial": info['serial'],
"wifi_strength": data['wifi_strength']
})
except Exception:
pass # IP is niet bereikbaar of geen HomeWizard plug
# Output de gevonden plugs als JSON
print(json.dumps(plugs))
# Start WiFi en scan
subnet = connect_wifi()
scan_network(subnet)
Thonny console output:
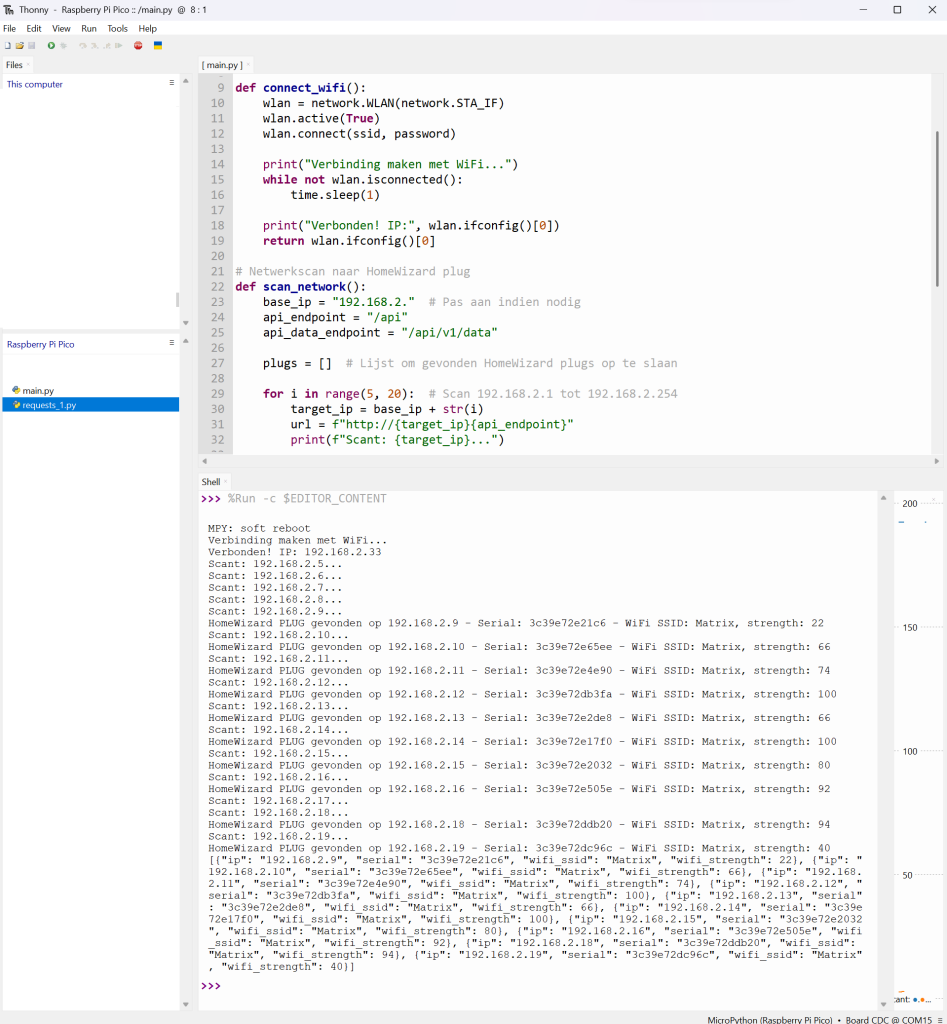