Table of Contents
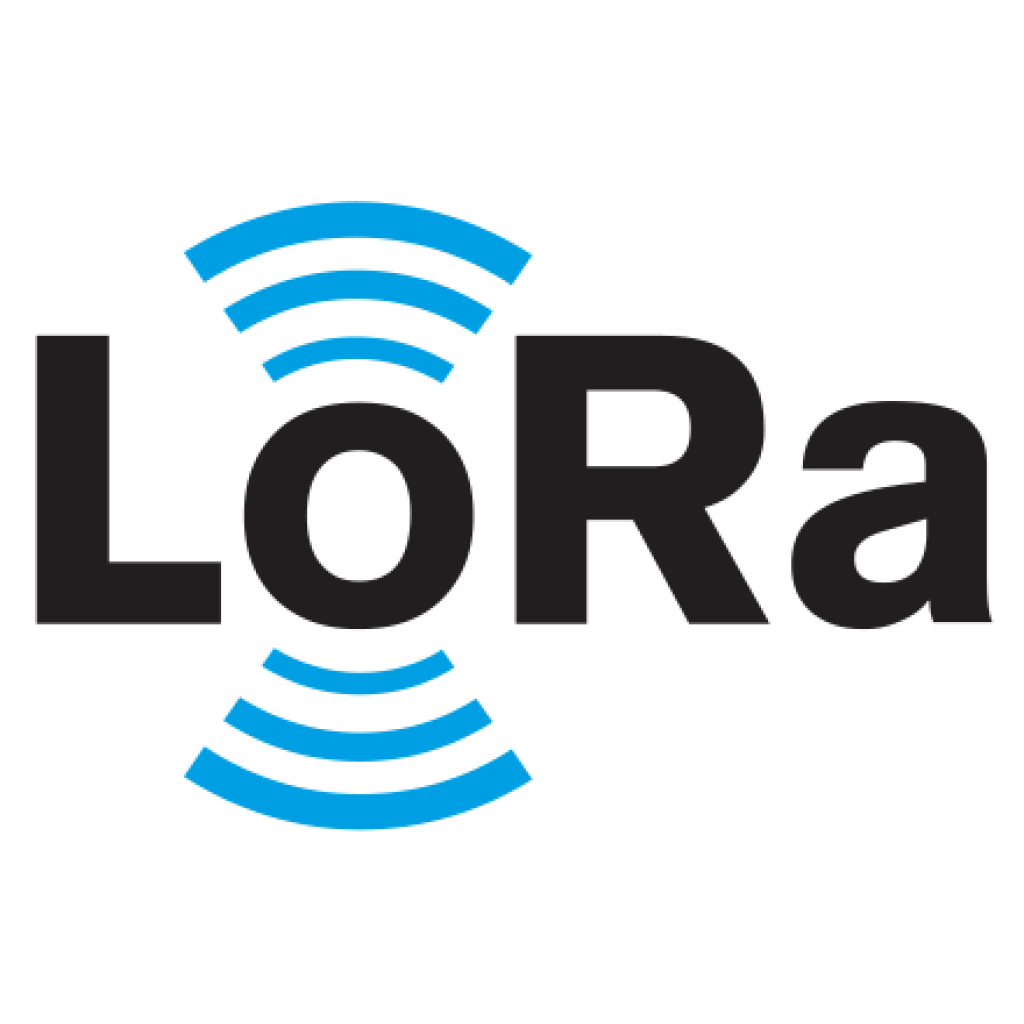
Introduction #
In this example we use a:
- Raspberry Pi as reciever
- Raspberry Pi Pico microcontroller as transmitter
Raspberry Pi as reciever #
Config RPI:
- Enable UART interface with raspi-config and reboot
- Install Python serial with: sudo apt-get install python3-serial
Wire Lora module:
RPI | LoRa Module |
---|---|
GND | GND |
3V3 | VCC |
GND | AUX |
TXD (GPIO 14 / PIN 8) | RXD |
RXD (GPIO 15 / PIN 10) | TXD |
GND | M1 |
GND | M0 |
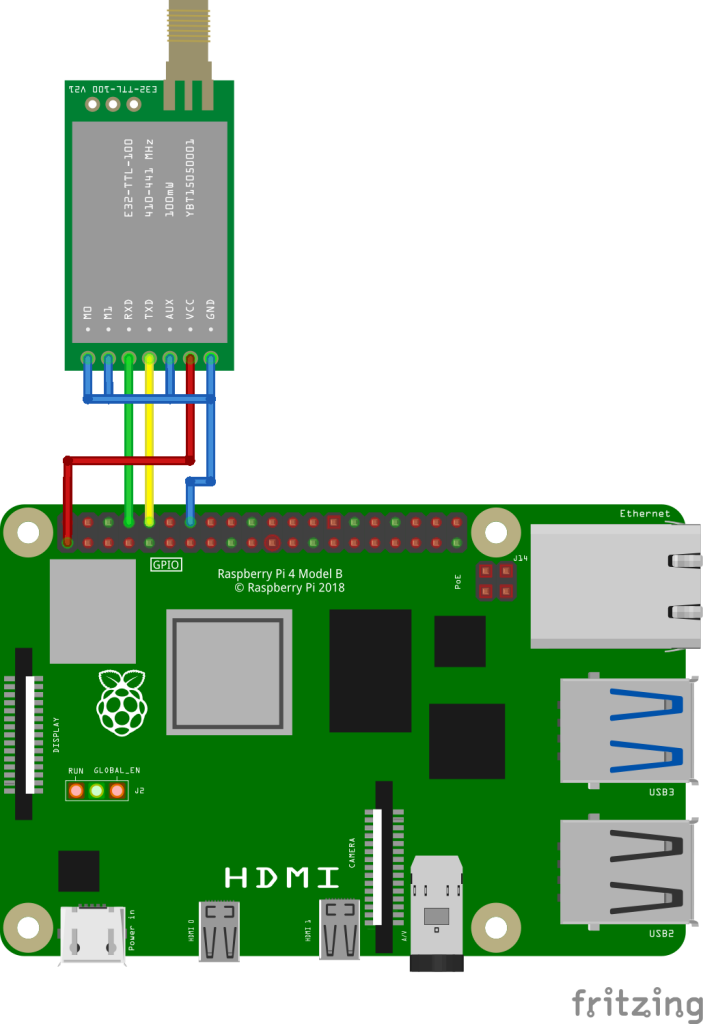
Python code:
import time
import serial
lora = serial.Serial('/dev/ttyS0', baudrate=9600, parity=serial.PARITY_NONE, stopbits=serial.STOPBITS_ONE, bytesize=serial.EIGHTBITS)
time.sleep(1)
try:
while True:
if lora.inWaiting() > 0:
print(lora.read())
except KeyboardInterrupt:
print("Exiting Program")
except:
print("Error, Exiting Program")
finally:
lora.close()
pass
Raspberry Pi PICO as transmitter #
Config RPI PICO:
- Install micropython on the PICO
Wire Lora module:
RPI PICO | LoRa Module |
---|---|
GND | GND |
3V3 | VCC |
GND | AUX |
TXD1 (GPIO 4 / PIN 6) | RXD |
RXD1 (GPIO 5 / PIN 7) | TXD |
GND | M1 |
GND | M0 |
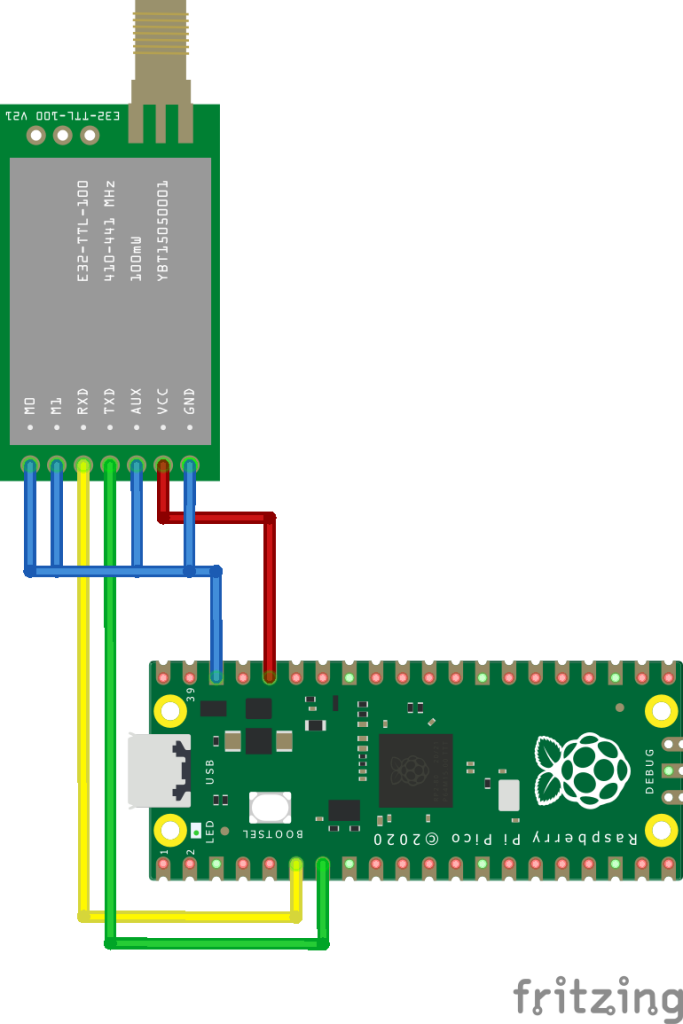
Python code:
import time
from machine import UART
lora = UART(1, 9600)
while True:
print("send message...")
lora.write("Hello World!")
time.sleep(2)
Recieving data #
When you have the PICO started and the Raspberry is listening with th epython script you will see this output on the rapsberry pi:
pi@raspberrypi:~ $ sudo python3 recieve.py
b'H'
b'e'
b'l'
b'l'
b'o'
b' '
b'W'
b'o'
b'r'
b'l'
b'd'
b'!'
Other examples #
Counter example on the transmiter:
import time
from machine import UART
lora = UART(1, 9600)
counter = 0
while True:
counter = counter + 1
print("send message:", counter)
lora.write(str(counter))
time.sleep(2)