Table of Contents
NTC Readout (micropython) #
We wire the circuit on the Breadboard and connect it to the Raspberry Pi Pico device.
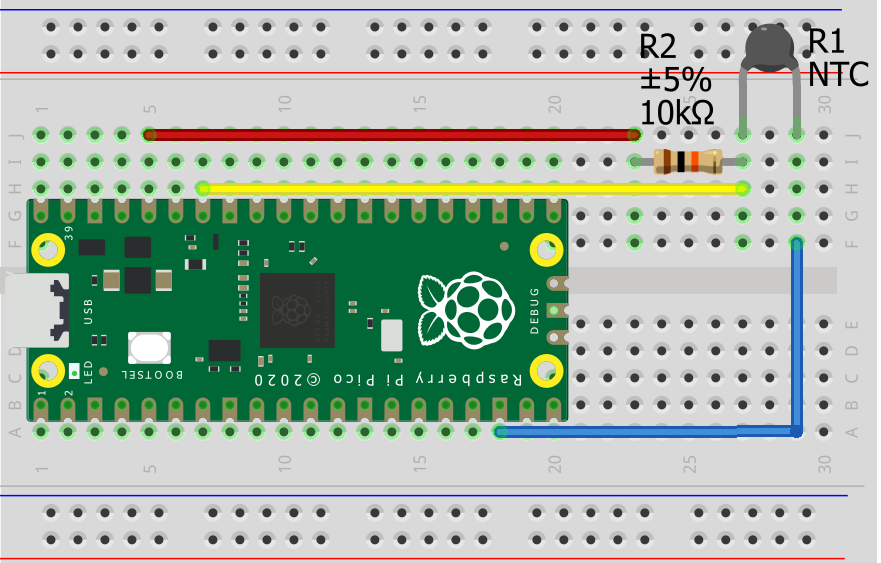
In the MicroPython code we need to do the following steps:
- We measure Vout using the ADC on Raspberry Pi Pico
- We calculate Rt using the Voltagee Divider Equation
- Then we use Steinhart-Hart equation for finding the Temperature
- Finally we convert from degrees Kelvin to Celsius
MicroPython Code Example #
from machine import ADC
from time import sleep
import math
adcpin = 28
thermistor = ADC(adcpin)
# Voltage Divider
Vin = 3.3
Ro = 10000 # 10k Resistor
# Steinhart Constants
A = 0.001129148
B = 0.000234125
C = 0.0000000876741
while True:
# Get Voltage value from ADC
adc = thermistor.read_u16() >> 4 # Get original 12-bit value 0-4096
print ("ADC Value:", adc)
Vout = (3.3/4096) * adc
# Calculate Resistance
Rt = (Vout * Ro) / (Vin - Vout)
#Rt = 10000 # Used for Testing. Setting Rt=10k should give TempC=25
# Steinhart - Hart Equation
TempK = 1 / (A + (B * math.log(Rt)) + C * math.pow(math.log(Rt), 3))
# Convert from Kelvin to Celsius
TempC = TempK - 273.15
#Correction for %5
TempC = TempC - 7
print(round(TempC, 1))
sleep(1)
Micropython code using ADS1115 and NTC 50K 3950 #
(NTC connected to channel 0 on ADS 1115 with voltage divider)
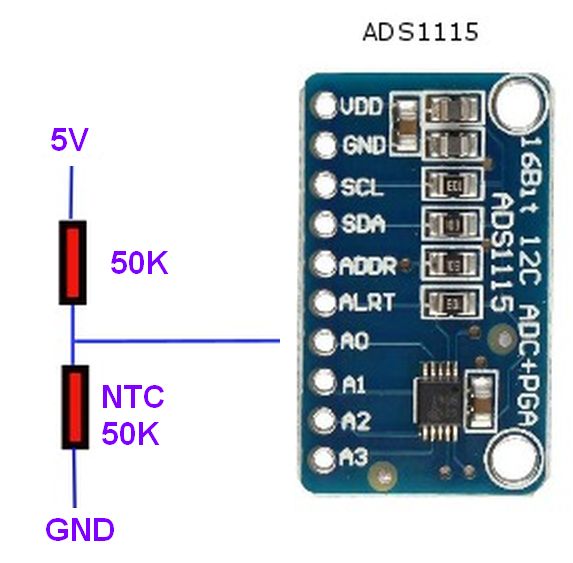
from machine import I2C, Pin
from time import sleep
from ads1x15 import ADS1115
import math
i2c=I2C(0, sda=Pin(8), scl=Pin(9))
adc = ADS1115(i2c, address=0x48, gain=0)
def calculate_temperature(adc_value):
# ADC Parameters
ADCvccpow = 5.0
ADCvccmax = 6.144 # ads1115 gain 0
ADCmaxvalue = 32768 # signed 16-bit
# Voltage Divider
R1 = 50000 # Pull-up resistor
# NTC Parameters
Rntc = 50000 # Resistance at 25°C (Ohms)
Beta = 3950 # Beta coefficient
Tnom = 25 # Nominal temperature in Celsius
T0 = 273.15 + Tnom # Convert 25°C to Kelvin
Vout = (ADCvccmax / ADCmaxvalue) * adc_value # Get Voltage value from ADC
#print(f"Vout: {Vout:.3f} V") # Debugging print statement
# Prevent division by zero or negative resistance
if Vout <= 0 or Vout >= ADCvccpow:
return None # Ongeldige meting
R_ntc = R1 * (ADCvccpow / Vout - 1) # Calculate Resistance
#print(f"R_ntc: {R_ntc:.1f} Ohm") # Debugging print statement
# Prevent log errors
if R_ntc <= 0:
return None # Ongeldige waarde
TempK = 1 / ((1 / T0) + (-1 / Beta) * math.log(R_ntc / Rntc)) # Calculate temperature using the Steinhart-Hart equation
TempC = TempK - 273.15 # Convert from Kelvin to Celsius
return round(TempC, 2)
while True:
adc_value = adc.read(0, 0)
temperature = calculate_temperature(adc_value)
print(f"Temperatuur: {temperature} °C")
sleep(.5)
Products #
Sources #
https://halvorsen.blog/documents/technology/iot/pico/pico_thermisor.php