Button interfacing technqiues #
Button can be interfaced with Pi Pico in two ways, pull-up configuration and pull down configuration. However since pi pico provides internal pull up configuration we are going to use that. In the pull up configuration, we will be receiving HIGH value at digital pin when button is not pushed and when the button is pushed we get the LOW value.
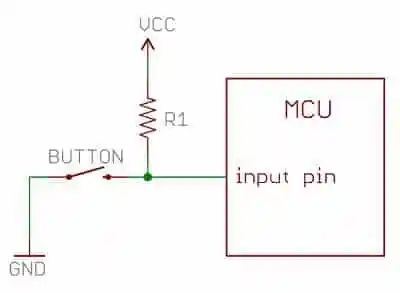
Code to read a button state (micropython) #
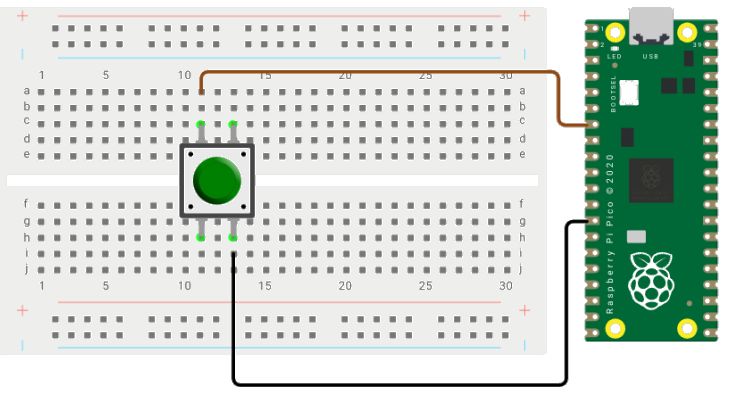
As can be seen, in the figure that Pi Ground pin is connected to one pin of button and the other pin of button is connected to GP5. We will use the internal pull-up configuration of pi pico.
from machine import Pin
import time
push_button = Pin(5, Pin.IN, Pin.PULL_UP)
while True:
button_state = push_button.value()
print(button_state)
time.sleep(.2)
Code to read a button state with led toggle (micropython) #
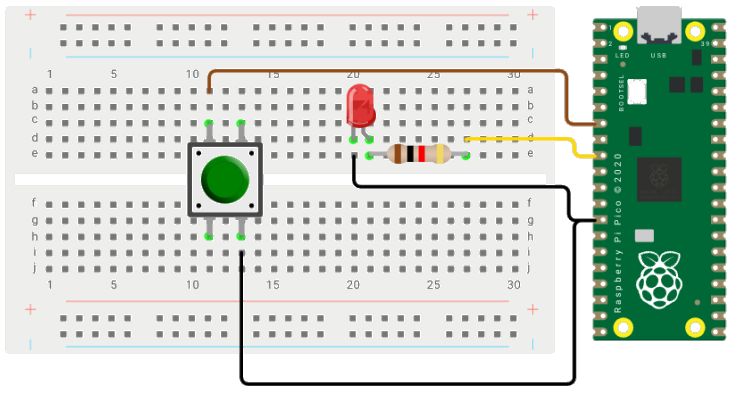
The anode of LED is connected to GP6 and cathode of LED is connected to GND.
from machine import Pin
from time import sleep
buttonPin = 5
LEDPin = 6
button_state = 0
led_state = 0
led = Pin(LEDPin, Pin.OUT)
push_button = Pin(buttonPin, Pin.IN, Pin.PULL_UP)
while True:
last_State = button_state
button_state = push_button.value()
if button_state == 0 and last_State == 1:
led_state = not(led_state)
led.value(led_state)
sleep(0.1)
source #
https://highvoltages.co/tutorial/pi-pico/button-with-pi-pico