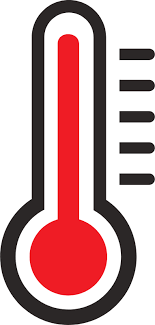
Reading internal temperature #
The Raspberry Pi Pico internal temperature sensor works by checking the voltage of a special diode connected to ADC4 on the Raspberry Pi Pico.
According to the datasheet, when it’s 27 degrees Celsius, the voltage is around 0.706V, and for every degree the temperature changes, the voltage goes down by about 1.721mV.
To find the temperature in Celsius using the voltage, you can use this formula:
T = 27 - (ADC_voltage - 0.706)/0.001721
Accurate temperature measurements with this sensor can be challenging due to variations in voltage and slope between devices. Consider it more as a reference tool than a precise measurement device. You may need to make adjustments for accuracy.
from machine import ADC
# Internal temperature sensor is connected to ADC channel 4
temp_sensor = ADC(4)
def read_internal_temperature():
# Read the raw ADC value
adc_value = temp_sensor.read_u16()
# Convert ADC value to voltage
voltage = adc_value * (3.3 / 65535.0)
# Temperature calculation based on sensor characteristics
temperature_celsius = 27 - (voltage - 0.706) / 0.001721
return temperature_celsius
def celsius_to_fahrenheit(temp_celsius):
temp_fahrenheit = temp_celsius * (9/5) + 32
return temp_fahrenheit
# Reading and printing the internal temperature
temperatureC = read_internal_temperature()
temperatureF = celsius_to_fahrenheit(temperatureC)
print("Internal Temperature:", temperatureC, "°C")
print("Internal Temperature:", temperatureF, "°F")