Table of Contents
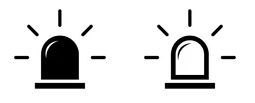
Code to blink internal led on Pico (mircopython) #
from machine import Pin
from time import sleep
led = Pin(25, Pin.OUT)
while True:
led.toggle()
sleep(0.5)
Code to blink internal led on Pico W (mircopython) #
Unlike the original Raspberry Pi Pico, the on-board LED on Pico W is not connected to a pin on RP2040, but instead to a GPIO pin on the wireless chip. MicroPython has been modified accordingly.
from machine import Pin
from time import sleep
led = Pin("LED", machine.Pin.OUT)
while True:
led.toggle()
sleep(0.5)
Code to blink a led on GPIO 28 with toggle (mircopython) #
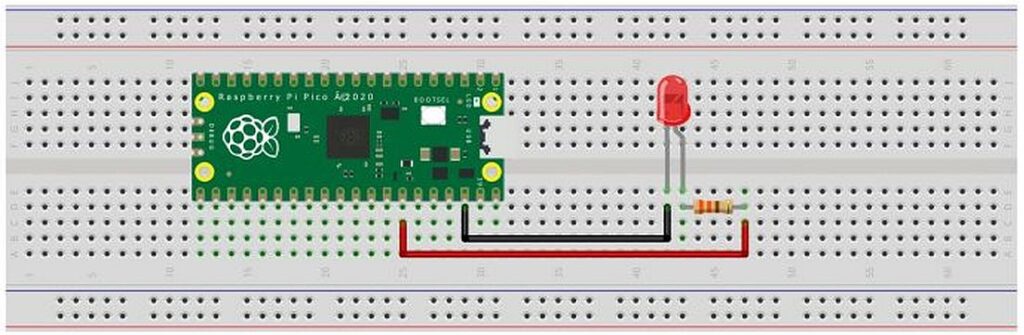
from machine import Pin
from time import sleep
led = Pin(28, Pin.OUT)
while True:
led.toggle()
sleep(0.5)
Code to blink a led on GPIO 28 with value (mircopython) #
from machine import Pin
from time import sleep
led = Pin(2, Pin.OUT)
while True:
led.value(1)
sleep(.2)
led.value(0)
sleep(.2)