Table of Contents
Using the Pico W as a Web Server #
This program turns your Pico W into a small web server. The web page has two links on it. One link will turn the on-board LED on and the other link will turn the LED off.
import network
import machine
import time
import ubinascii
import socket
onboard_led = machine.Pin("LED", machine.Pin.OUT)
onboard_led.value(0) # LED off
# Fill in your WiFi network name (ssid) and password here:
wifi_ssid = ""
wifi_password = ""
# Connect to WiFi
print('Connecting to WiFi Network Name:', wifi_ssid)
wlan = network.WLAN(network.STA_IF)
wlan.config(hostname="RPI_PICOW")
wlan.active(True) # power up the WiFi chip
print('Waiting for wifi chip to power up...')
time.sleep(3) # wait three seconds for the chip to power up and initialize
wlan.connect(wifi_ssid, wifi_password)
while wlan.isconnected() == False:
print('Waiting for connection...')
time.sleep(1)
print("Success! We have connected to your access point!")
print("Try to ping the device at", wlan.ifconfig()[0])
print("- subnetmask:", wlan.ifconfig()[1])
print("- gateway:", wlan.ifconfig()[2])
mac = ubinascii.hexlify(wlan.config('mac'),':').decode()
print("- MAC address:", mac)
addr = socket.getaddrinfo('0.0.0.0', 80)[0][-1]
print('addr:', addr)
s = socket.socket() # Open socket
s.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
s.bind(addr)
s.listen(1)
print('listening on', addr)
html = """<!DOCTYPE html>
<html>
<head>
<title>Web Server On Pico W</title>
</head>
<body>
<h1>Pico Wireless Web Server</h1>
<p>%s</p>
<a href="/light/on">Turn On</a>
<a href="/light/off">Turn Off</a>
</body>
</html>
"""
stateis = "LED is OFF"
# Listen for connections
while True:
try:
cl, addr = s.accept()
print('client connected from', addr)
request = cl.recv(1024)
print(request)
request = str(request)
led_on = request.find('/light/on')
led_off = request.find('/light/off')
print( 'led on = ' + str(led_on))
print( 'led off = ' + str(led_off))
if led_on == 6:
print("led on")
onboard_led.value(1)
stateis = "LED is ON"
if led_off == 6:
print("led off")
onboard_led.value(0)
stateis = "LED is OFF"
# generate the we page with the stateis as a parameter
response = html % stateis
cl.send('HTTP/1.0 200 OK\r\nContent-type: text/html\r\n\r\n')
cl.send(response)
cl.close()
except OSError as e:
cl.close()
print('connection closed')
Result
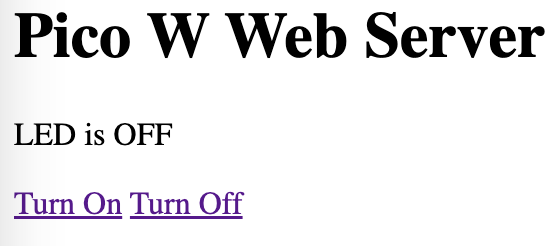
Using the Pico W as a Web Server with buttons and temperature #
import network
import machine
import time
import ubinascii
import socket
from machine import ADC
# Internal temperature sensor is connected to ADC channel 4
temp_sensor = ADC(4)
onboard_led = machine.Pin("LED", machine.Pin.OUT)
onboard_led.value(0) # LED off
# Fill in your WiFi network name (ssid) and password here:
wifi_ssid = "Matrix"
wifi_password = "139726845$e"
# Connect to WiFi
print('Connecting to WiFi Network Name:', wifi_ssid)
wlan = network.WLAN(network.STA_IF)
wlan.config(hostname="RPI_PICOW")
wlan.active(True) # power up the WiFi chip
print('Waiting for wifi chip to power up...')
time.sleep(3) # wait three seconds for the chip to power up and initialize
wlan.connect(wifi_ssid, wifi_password)
while wlan.isconnected() == False:
print('Waiting for connection...')
time.sleep(1)
print("Success! We have connected to your access point!")
ip = wlan.ifconfig()[0]
print("Try to ping the device at", ip)
print("- subnetmask:", wlan.ifconfig()[1])
print("- gateway:", wlan.ifconfig()[2])
mac = ubinascii.hexlify(wlan.config('mac'),':').decode()
print("- MAC address:", mac)
# Open a socket
address = (ip, 80)
connection = socket.socket()
connection.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
connection.bind(address)
connection.listen(1)
print(connection)
def webpage(temperature, state):
#Template HTML
html = f"""
<!DOCTYPE html>
<html>
<form action="./lighton">
<input type="submit" value="Light on" />
</form>
<form action="./lightoff">
<input type="submit" value="Light off" />
</form>
<p>LED is {state}</p>
<p>Pico temperature is: {temperature} degrees celcius</p>
</body>
</html>
"""
return str(html)
# Listen for connections
def serve(connection):
#Start a web server
state = 'OFF'
onboard_led.value(0)
while True:
client = connection.accept()[0]
request = client.recv(1024)
request = str(request)
try:
request = request.split()[1]
except IndexError:
pass
if request == '/lighton?':
onboard_led.value(1)
state = 'ON'
elif request =='/lightoff?':
onboard_led.value(0)
state = 'OFF'
temperature = read_internal_temperature()
html = webpage(temperature, state)
client.send(html)
client.close()
def read_internal_temperature():
# Read the raw ADC value
adc_value = temp_sensor.read_u16()
# Convert ADC value to voltage
voltage = adc_value * (3.3 / 65535.0)
# Temperature calculation based on sensor characteristics
temperature_celsius = 27 - (voltage - 0.706) / 0.001721
return temperature_celsius
def celsius_to_fahrenheit(temp_celsius):
temp_fahrenheit = temp_celsius * (9/5) + 32
return temp_fahrenheit
try:
serve(connection)
except KeyboardInterrupt:
machine.reset()
Result
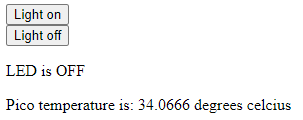
Products #
Source #
https://www.coderdojotc.org/micropython/basics/06-wireless
https://www.instructables.com/Raspberry-Pi-Pico-W-Web-Server