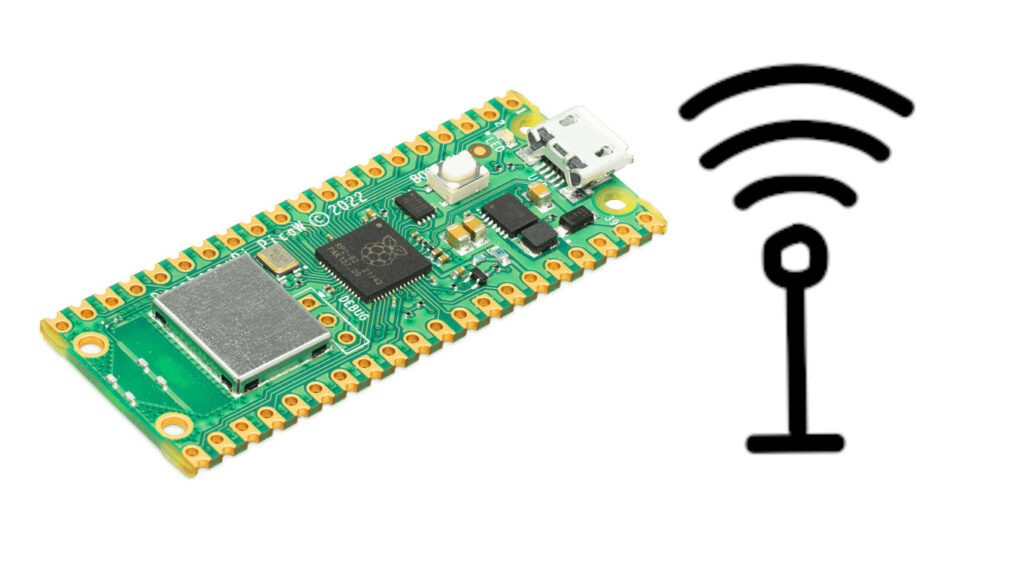
Connect to WLAN #
Passwords need to be kept securely and privately. In this step, you will add your WiFi password into your Python file. Make sure you don’t share your file with anyone that you wouldn’t want to tell your password to.
To connect to a WiFi network, you will need to know your service set identifier (SSID). This is the name of your WiFi network. You will also need your WiFi password. These can usually be found written on your wireless router, although you should have changed the default password to something unique.
import network
import machine
import time
import ubinascii
onboard_led = machine.Pin("LED", machine.Pin.OUT)
onboard_led.value(0) # LED off
# Fill in your WiFi network name (ssid) and password here:
wifi_ssid = ""
wifi_password = ""
# Connect to WiFi
print('Connecting to WiFi Network Name:', wifi_ssid)
wlan = network.WLAN(network.STA_IF)
wlan.config(hostname="RPI_PICOW")
wlan.active(True) # power up the WiFi chip
print('Waiting for wifi chip to power up...')
time.sleep(3) # wait three seconds for the chip to power up and initialize
wlan.connect(wifi_ssid, wifi_password)
while wlan.isconnected() == False:
print('Waiting for connection...')
time.sleep(1)
onboard_led.value(1)
print("Success! We have connected to your access point!")
print("Try to ping the device at", wlan.ifconfig()[0])
print("- subnetmask:", wlan.ifconfig()[1])
print("- gateway:", wlan.ifconfig()[2])
mac = ubinascii.hexlify(wlan.config('mac'),':').decode()
print("- MAC address:", mac)
Output:
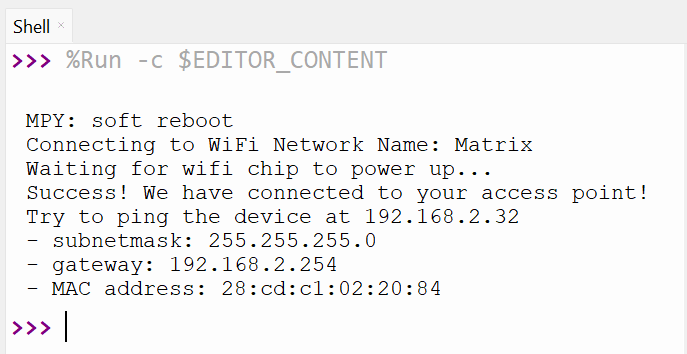
The Raspberry Pi Pico W won’t connect #
- Make sure that you are using the correct SSID and password.
- If you are on a school or work WLAN, unauthorised devices might not be permitted access to the WiFi.
- Unplug your Raspberry Pi Pico W from your computer to power it off, then plug it back in. This can be a problem when you have connected once, and then try to connect again.
Using WIFI secrets.py #
By convention, we put both our SSID and password in a python file called “secrets.py”. This file should never be checked into a public source code repository. We can add secrets.py
to the .gitignore file to make sure the secrets.py is never checked into GitHub and exposing your passwords to everyone.
SSID = "MY_WIFI_NETWORK_NAME"
PASSWORD = "MY_WIFI_PASSWORD"
By importing the secrets.py file you can then reference your network name like this:
import secrets
...
print('Connecting to WiFi Network Name:', secrets.SSID)
...
Testing HTTP GET #
To test HTT GET you need to import the library: urequests, example
import urequests
...
...
astronauts = urequests.get("http://api.open-notify.org/astros.json").json()
print(astronauts)
...
Output
{'message': 'success', 'people': [
{'name': 'Oleg Kononenko', 'craft': 'ISS'},
{'name': 'Nikolai Chub', 'craft': 'ISS'},
{'name': 'Tracy Caldwell Dyson', 'craft': 'ISS'},
{'name': 'Matthew Dominick', 'craft': 'ISS'},
{'name': 'Michael Barratt', 'craft': 'ISS'},
{'name': 'Jeanette Epps', 'craft': 'ISS'},
{'name': 'Alexander Grebenkin', 'craft': 'ISS'},
{'name': 'Butch Wilmore', 'craft': 'ISS'},
{'name': 'Sunita Williams', 'craft': 'ISS'},
{'name': 'Li Guangsu', 'craft': 'Tiangong'},
{'name': 'Li Cong', 'craft': 'Tiangong'},
{'name': 'Ye Guangfu', 'craft': 'Tiangong'}
], 'number': 12}
Or a bit nicer (total code)
import network
import machine
import time
import ubinascii
import urequests
onboard_led = machine.Pin("LED", machine.Pin.OUT)
onboard_led.value(0) # LED off
# Fill in your WiFi network name (ssid) and password here:
wifi_ssid = ""
wifi_password = ""
# Connect to WiFi
print('Connecting to WiFi Network Name:', wifi_ssid)
wlan = network.WLAN(network.STA_IF)
wlan.config(hostname="RPI_PICOW")
wlan.active(True) # power up the WiFi chip
print('Waiting for wifi chip to power up...')
time.sleep(3) # wait three seconds for the chip to power up and initialize
wlan.connect(wifi_ssid, wifi_password)
while wlan.isconnected() == False:
print('Waiting for connection...')
time.sleep(1)
onboard_led.value(1)
print("Success! We have connected to your access point!")
print("Try to ping the device at", wlan.ifconfig()[0])
print("- subnetmask:", wlan.ifconfig()[1])
print("- gateway:", wlan.ifconfig()[2])
mac = ubinascii.hexlify(wlan.config('mac'),':').decode()
print("- MAC address:", mac)
astronauts = urequests.get("http://api.open-notify.org/astros.json").json()
number = astronauts['number']
print('There are', number, 'astronauts in space.')
for i in range(number):
print(i+1, astronauts['people'][i]['name'])
Output:
There are 12 astronauts in space.
1 Oleg Kononenko
2 Nikolai Chub
3 Tracy Caldwell Dyson
4 Matthew Dominick
5 Michael Barratt
6 Jeanette Epps
7 Alexander Grebenkin
8 Butch Wilmore
9 Sunita Williams
10 Li Guangsu
11 Li Cong
12 Ye Guangfu
Source #
https://projects.raspberrypi.org/en/projects/get-started-pico-w/2
https://www.coderdojotc.org/micropython/basics/06-wireless