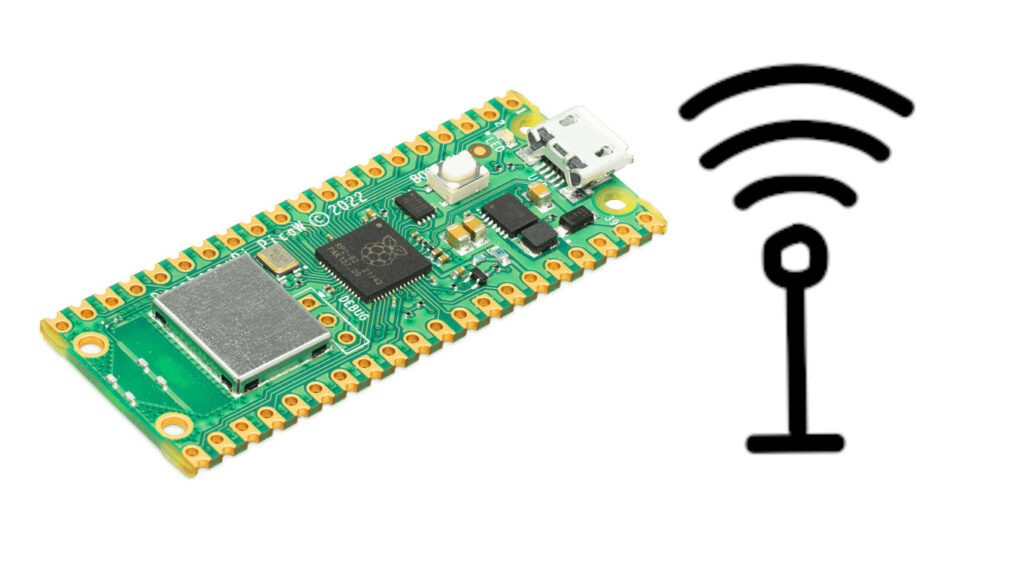
Mycropython – Connect to WLAN with onboarding #
The script process is as follows:
- Pico WiFi start as access point (with SSID added from pico MAC)
- User connects with Pico WiFi
- User goes to 192.168.4.1
- User selects network and fills in password of that network
- Settings are saved in a config file
- Pico reboots and connect to WiFi stored in the settings config.
import network
import socket
import ubinascii
import time
import json
import machine
SSID_PREFIX = "pico-"
CONFIG_FILE = "config.json"
def load_config():
try:
with open(CONFIG_FILE, 'r') as f:
config = json.load(f)
return config
except Exception as e:
print(f"Configuratie niet gevonden: {e}")
return {'ssid': '', 'password': '', 'wificonfig': 'incomplete'}
def start_ap():
mac = ubinascii.hexlify(network.WLAN().config('mac'), ':').decode().replace(':', '')
ssid = SSID_PREFIX + mac
ap = network.WLAN(network.AP_IF)
ap.active(True)
ap.config(essid=ssid, password="12345678") # Simpele standaard wachtwoord
print(f"AP gestart: {ssid}")
while not ap.active():
time.sleep(0.5)
print("AP actief")
return ap
def connect_wifi(ssid, password):
wlan = network.WLAN(network.STA_IF)
wlan.active(True)
wlan.connect(ssid, password)
print(f"Verbinden met {ssid}...")
for _ in range(20):
if wlan.isconnected():
print("Wi-Fi verbonden!")
print("IP-adres:", wlan.ifconfig()[0])
return True
time.sleep(1)
print("Wi-Fi verbinding mislukt")
return False
def scan_networks():
wlan = network.WLAN(network.STA_IF)
wlan.active(True)
print("Scanning...")
networks = wlan.scan()
return [n[0].decode('utf-8') for n in networks]
def web_page(networks):
options = ''.join([f'<option>{ssid}</option>' for ssid in networks])
html = f"""
<html>
<body>
<h1>Pico Wi-Fi Config</h1>
<form action="/" method="POST">
<label>Wi-Fi Netwerk:</label>
<select name="ssid">{options}</select><br>
<label>Wachtwoord:</label>
<input type="text" name="password"><br>
<input type="submit" value="Verbind">
</form>
</body>
</html>
"""
return html
def save_config(ssid, password):
with open(CONFIG_FILE, 'w') as f:
json.dump({'ssid': ssid, 'password': password, 'wificonfig': 'complete'}, f)
print(f"Configuratie opgeslagen: {ssid}")
def url_replace(text):
replacements = {
'%24': '$',
'%20': ' ',
'%40': '@',
'%3A': ':',
'%2F': '/',
'%2B': '+',
'%3D': '='
}
for k, v in replacements.items():
text = text.replace(k, v)
return text
def webserver(ap):
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.bind(('0.0.0.0', 80))
s.listen(5)
print("Webserver gestart")
while True:
conn, addr = s.accept()
print(f"Verbonden met {addr}")
request = conn.recv(1024).decode()
print(request)
if 'POST' in request:
params = request.split('\r\n')[-1]
ssid = params.split('ssid=')[1].split('&')[0]
password = url_replace(params.split('password=')[1])
save_config(ssid, password)
conn.send('HTTP/1.1 200 OK\nContent-Type: text/html\n\nInstellingen opgeslagen! Herstart...')
time.sleep(3)
machine.reset()
else:
networks = scan_networks()
response = web_page(networks)
conn.send('HTTP/1.1 200 OK\nContent-Type: text/html\n\n' + response)
conn.close()
config = load_config()
if config['wificonfig'] == 'complete':
if not connect_wifi(config['ssid'], config['password']):
print("Geen Wi-Fi verbinding mogelijk, terug naar AP mode")
ap = start_ap()
webserver(ap)
else:
ap = start_ap()
webserver(ap)
Step 1 – acces point started
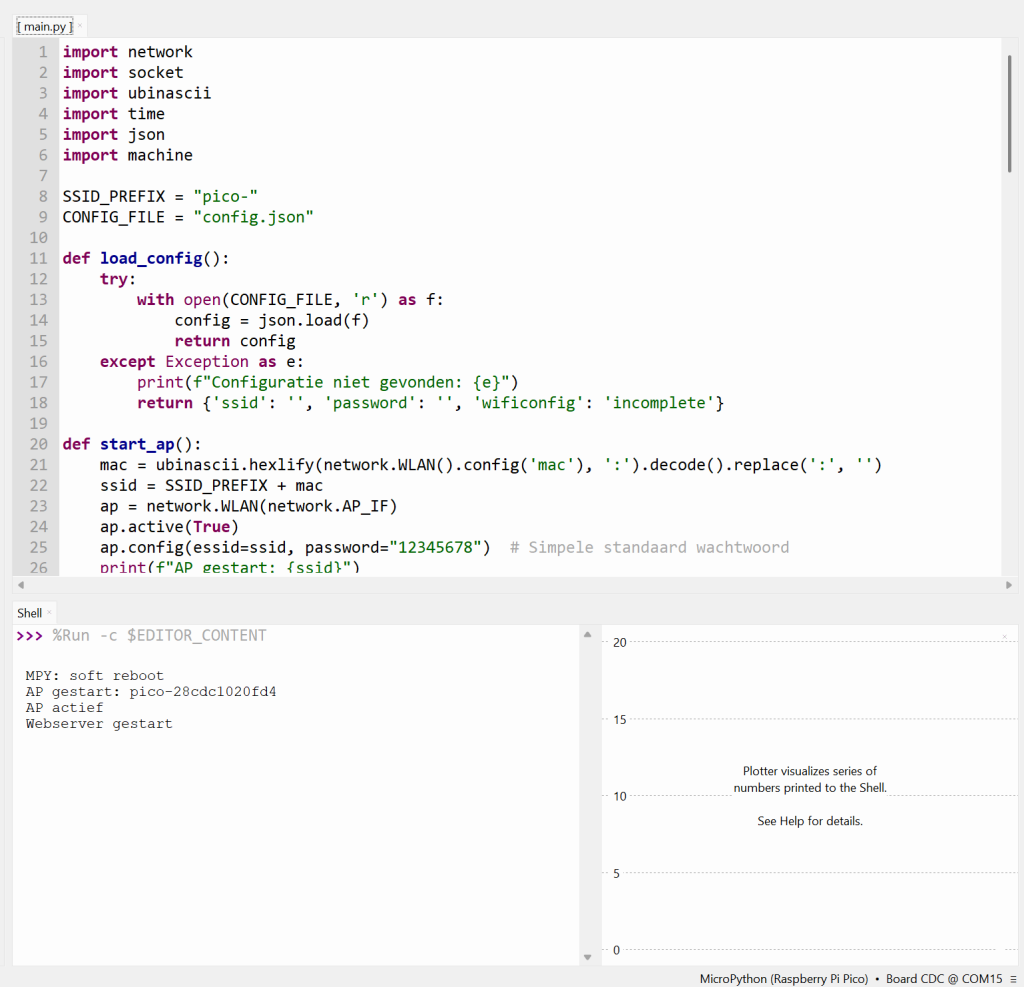
Step 2 – Connect to Pico WiFi from a mobile device
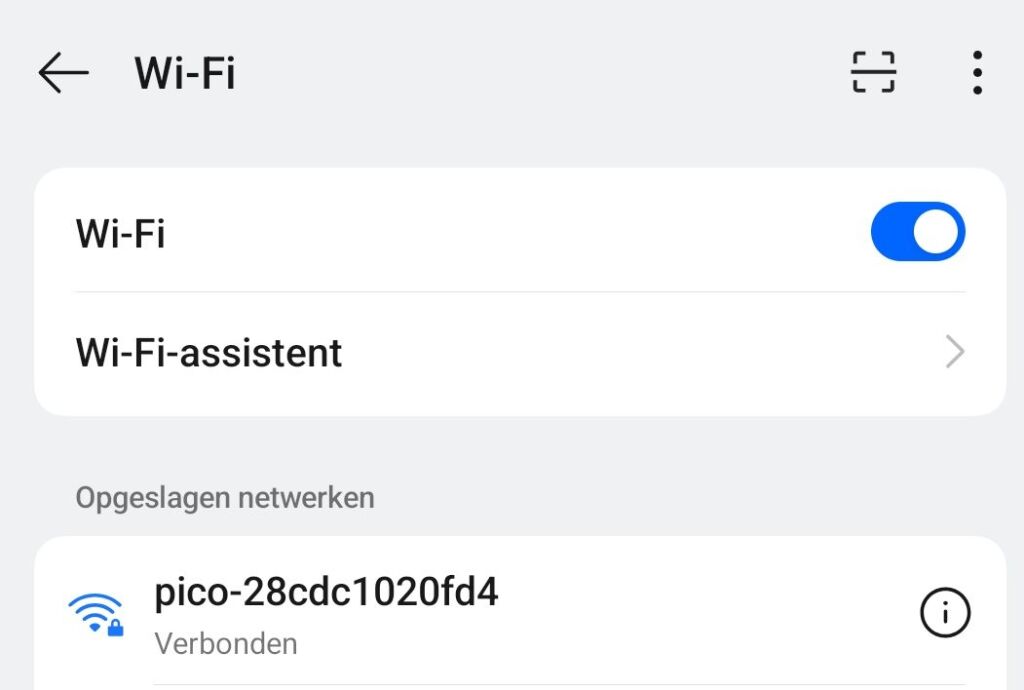
Step 3 – Go to 192.168.4.1 select network, enter password
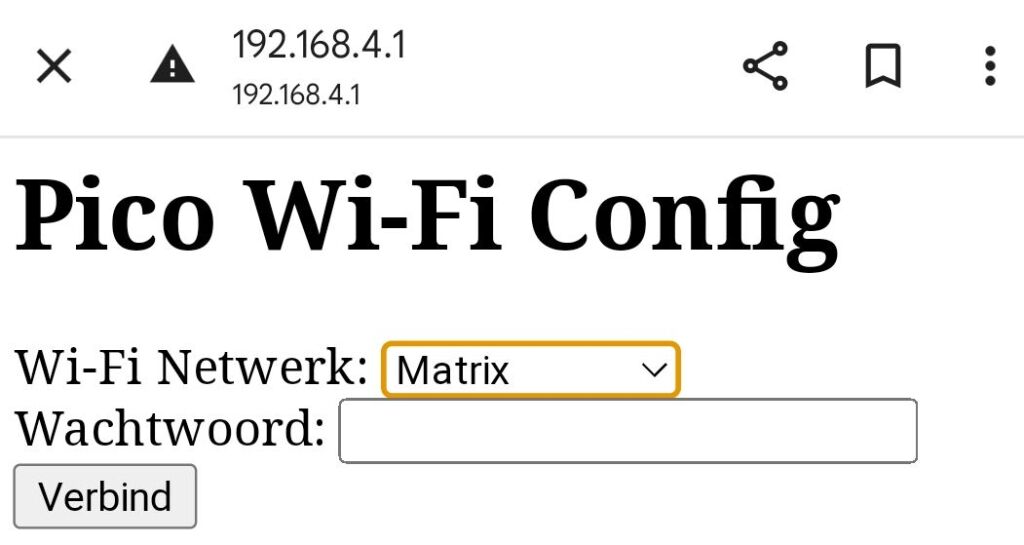
In Thonny you will see the output of connection made to the Pico
Verbonden met ('192.168.4.16', 49446)
GET / HTTP/1.1
User-Agent: Dalvik/2.1.0 (Linux; U; Android 15; CPH2465 Build/UKQ1.230924.001)
Host: 192.168.4.1
Connection: Keep-Alive
Accept-Encoding: gzip
Step 4 – Settings write and reboot
Settings are now saved in the file config.json, JSON contents:
{"wificonfig": "complete", "password": "PASSWORD", "ssid": "Matrix"}
After reboot you will see the connection to your WiFi network in the console:
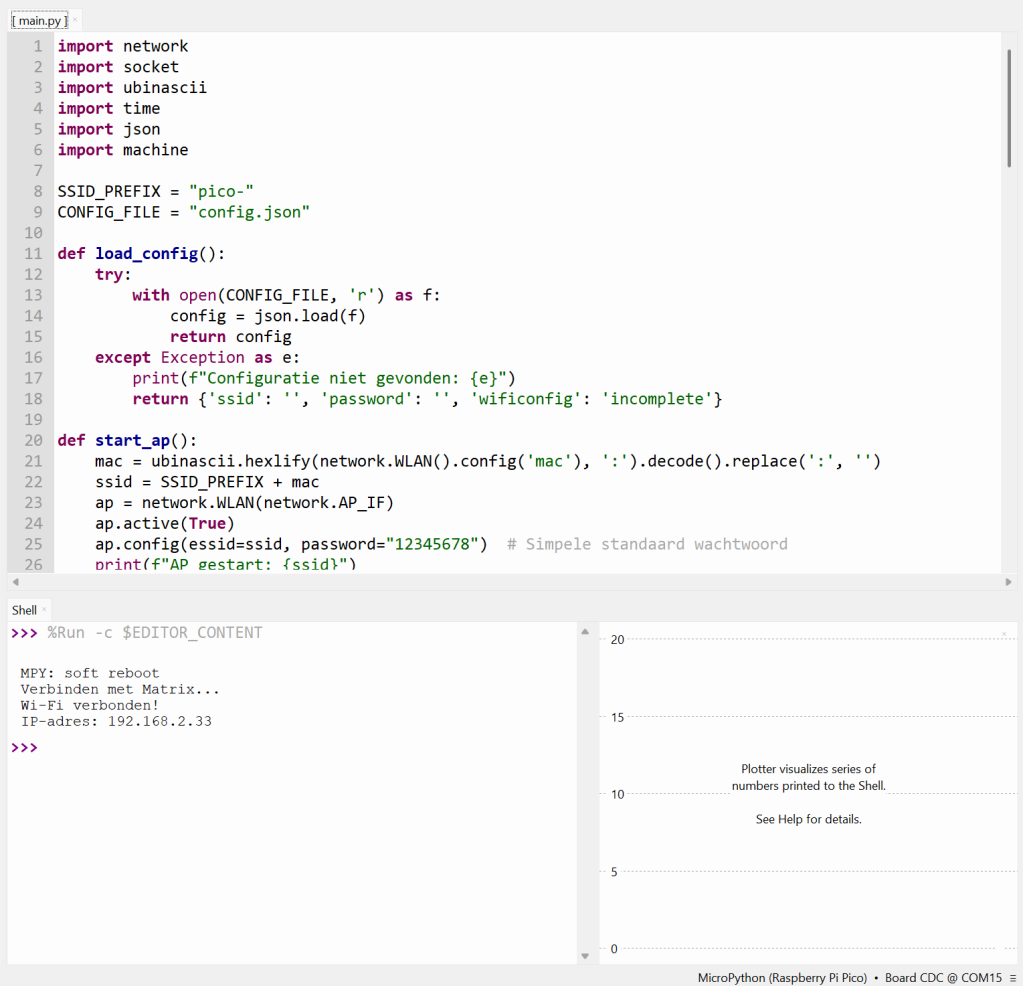
Other station IP then 192.168.4.1 #
I have found a topic wich could let you choose another IP then the default 192.168.4.1 in station mode.
Conclusions I have come to:
DHCP assignment of IP addresses is flawed, connecting devices need to use static addresses.
The interface always starts up with 192.168.4.1 but can be changed and works with the change.
Setting of the SSID & Password must be done before activating the interface.
A small delay is necessary in starting and stopping the interface.
The previous IP address is remembered between program starts but not after power off.
Program code and shell output:
import time
import network
from SSID_AP import SSID_AP
IP = '192.168.12.1'
SUBNET = '255.255.255.0'
GATEWAY = '192.168.12.1'
DNS = '0.0.0.0'
ssid = SSID_AP['ssid']
pw = SSID_AP['pw']
ap = network.WLAN(network.AP_IF)
ap.config(ssid=ssid, password=pw)
ap.active(True)
time.sleep(0.1)
print('1',ap)
ap.ifconfig((IP,SUBNET,GATEWAY,DNS))
time.sleep(0.1)
print('3',ap)
while True:
pass
>>> %Run -c $EDITOR_CONTENT
1 <CYW43 AP up 192.168.4.1>
3 <CYW43 AP up 192.168.12.1>