Table of Contents
Schematic #
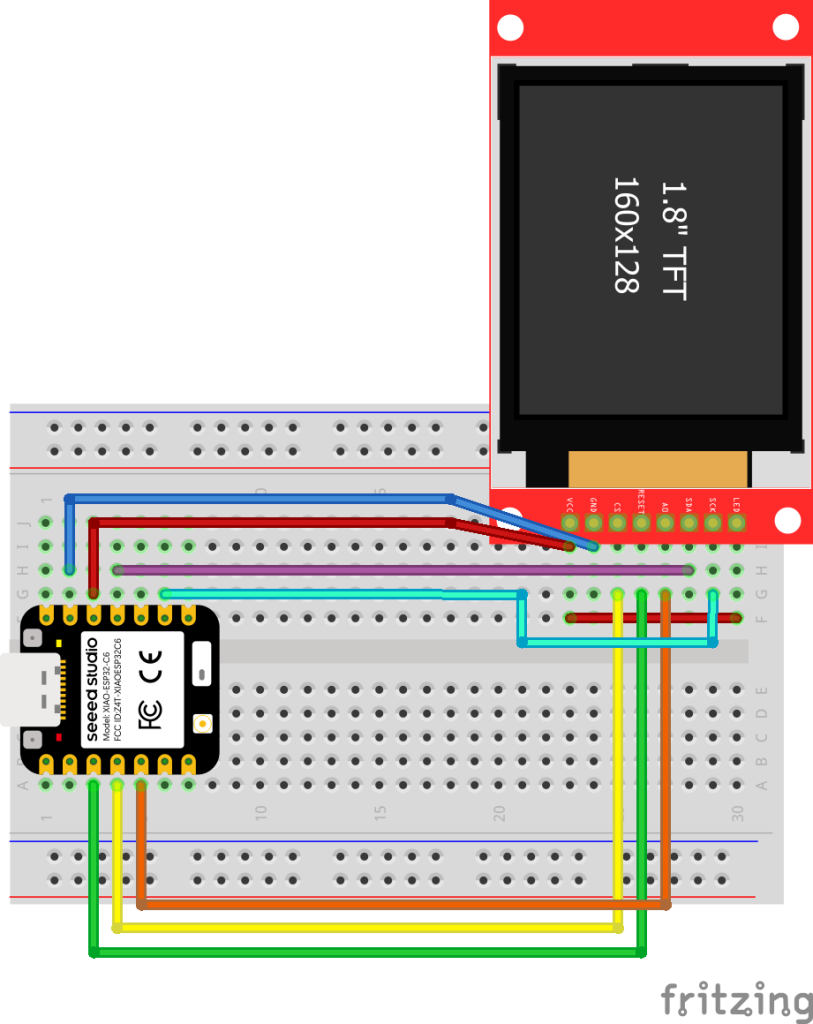
ESP32C6 - DISPLAY
GND - GND
VCC 3.3V - VCC, LED
D3/SS - CS
D2 - RESET
D4 - A0
D10 MOSI - SDA
D8 SCK - SCK
Testcode “rotation” using adafruit library #
Source: https://github.com/adafruit/Adafruit-ST7735-Library
Note: Please use the XIAO ESP32-C6 board for the pin definitions (not ESP32C6 Dev Module)
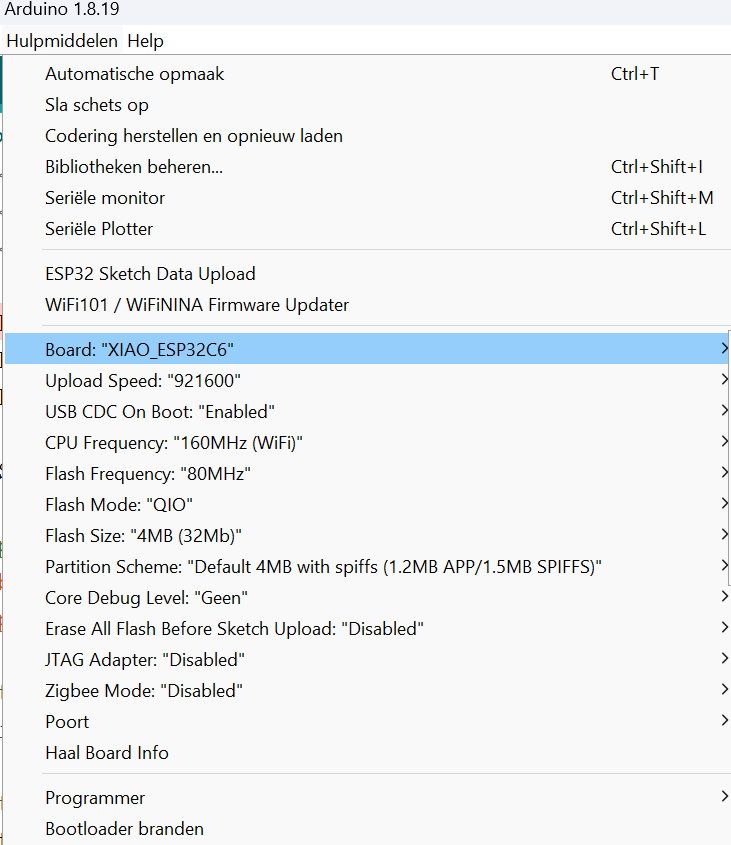
Code
#include <Adafruit_GFX.h> // Core graphics library
#include <Adafruit_ST7735.h> // Hardware-specific library for ST7735
#include <SPI.h>
#define TFT_CS D3 // 21
#define TFT_RST D2 // Of -1 als je de reset pin niet gebruikt
#define TFT_DC D4
Adafruit_ST7735 tft = Adafruit_ST7735(TFT_CS, TFT_DC, TFT_RST);
void setup(void) {
Serial.begin(9600);
Serial.print("Hello! Adafruit ST77XX rotation test");
// Use this initializer if you're using a 1.8" TFT
// tft.initR(INITR_BLACKTAB); // initialize a ST7735S chip, black tab
// Use this initializer (uncomment) if you're using a 1.44" TFT
tft.initR(INITR_144GREENTAB); // initialize a ST7735S chip, black tab
// Use this initializer (uncomment) if you're using a 0.96" 180x60 TFT
//tft.initR(INITR_MINI160x80); // initialize a ST7735S chip, mini display
// Use this initializer (uncomment) if you're using a 1.54" 240x240 TFT
//tft.init(240, 240); // initialize a ST7789 chip, 240x240 pixels
// OR use this initializer (uncomment) if using a 2.0" 320x240 TFT:
//tft.init(240, 320); // Init ST7789 320x240
// OR use this initializer (uncomment) if using a 1.14" 240x135 TFT:
//tft.init(135, 240); // Init ST7789 240x135
// SPI speed defaults to SPI_DEFAULT_FREQ defined in the library, you can override it here
// Note that speed allowable depends on chip and quality of wiring, if you go too fast, you
// may end up with a black screen some times, or all the time.
//tft.setSPISpeed(40000000);
Serial.println("init");
tft.setTextWrap(false); // Allow text to run off right edge
tft.fillScreen(ST77XX_BLACK);
Serial.println("This is a test of the rotation capabilities of the TFT library!");
Serial.println("Press <SEND> (or type a character) to advance");
}
void loop(void) {
rotateLine();
rotateText();
rotatePixel();
rotateFastline();
rotateDrawrect();
rotateFillrect();
rotateDrawcircle();
rotateFillcircle();
rotateTriangle();
rotateFillTriangle();
rotateRoundRect();
rotateFillRoundRect();
rotateChar();
rotateString();
}
void rotateText() {
for (uint8_t i=0; i<4; i++) {
tft.fillScreen(ST77XX_BLACK);
Serial.println(tft.getRotation(), DEC);
tft.setCursor(0, 30);
tft.setTextColor(ST77XX_RED);
tft.setTextSize(1);
tft.println("Hello World!");
tft.setTextColor(ST77XX_YELLOW);
tft.setTextSize(2);
tft.println("Hello World!");
tft.setTextColor(ST77XX_GREEN);
tft.setTextSize(3);
tft.println("Hello World!");
tft.setTextColor(ST77XX_BLUE);
tft.setTextSize(4);
tft.print(1234.567);
delay(500);
tft.setRotation(tft.getRotation()+1);
}
}
void rotateFillcircle(void) {
for (uint8_t i=0; i<4; i++) {
tft.fillScreen(ST77XX_BLACK);
Serial.println(tft.getRotation(), DEC);
tft.fillCircle(10, 30, 10, ST77XX_YELLOW);
delay(500);
tft.setRotation(tft.getRotation()+1);
}
}
void rotateDrawcircle(void) {
for (uint8_t i=0; i<4; i++) {
tft.fillScreen(ST77XX_BLACK);
Serial.println(tft.getRotation(), DEC);
tft.drawCircle(10, 30, 10, ST77XX_YELLOW);
delay(500);
tft.setRotation(tft.getRotation()+1);
}
}
void rotateFillrect(void) {
for (uint8_t i=0; i<4; i++) {
tft.fillScreen(ST77XX_BLACK);
Serial.println(tft.getRotation(), DEC);
tft.fillRect(10, 20, 10, 20, ST77XX_GREEN);
delay(500);
tft.setRotation(tft.getRotation()+1);
}
}
void rotateDrawrect(void) {
for (uint8_t i=0; i<4; i++) {
tft.fillScreen(ST77XX_BLACK);
Serial.println(tft.getRotation(), DEC);
tft.drawRect(10, 20, 10, 20, ST77XX_GREEN);
delay(500);
tft.setRotation(tft.getRotation()+1);
}
}
void rotateFastline(void) {
for (uint8_t i=0; i<4; i++) {
tft.fillScreen(ST77XX_BLACK);
Serial.println(tft.getRotation(), DEC);
tft.drawFastHLine(0, 20, tft.width(), ST77XX_RED);
tft.drawFastVLine(20, 0, tft.height(), ST77XX_BLUE);
delay(500);
tft.setRotation(tft.getRotation()+1);
}
}
void rotateLine(void) {
for (uint8_t i=0; i<4; i++) {
tft.fillScreen(ST77XX_BLACK);
Serial.println(tft.getRotation(), DEC);
tft.drawLine(tft.width()/2, tft.height()/2, 0, 0, ST77XX_RED);
delay(500);
tft.setRotation(tft.getRotation()+1);
}
}
void rotatePixel(void) {
for (uint8_t i=0; i<4; i++) {
tft.fillScreen(ST77XX_BLACK);
Serial.println(tft.getRotation(), DEC);
tft.drawPixel(10,20, ST77XX_WHITE);
delay(500);
tft.setRotation(tft.getRotation()+1);
}
}
void rotateTriangle(void) {
for (uint8_t i=0; i<4; i++) {
tft.fillScreen(ST77XX_BLACK);
Serial.println(tft.getRotation(), DEC);
tft.drawTriangle(20, 10, 10, 30, 30, 30, ST77XX_GREEN);
delay(500);
tft.setRotation(tft.getRotation()+1);
}
}
void rotateFillTriangle(void) {
for (uint8_t i=0; i<4; i++) {
tft.fillScreen(ST77XX_BLACK);
Serial.println(tft.getRotation(), DEC);
tft.fillTriangle(20, 10, 10, 30, 30, 30, ST77XX_RED);
delay(500);
tft.setRotation(tft.getRotation()+1);
}
}
void rotateRoundRect(void) {
for (uint8_t i=0; i<4; i++) {
tft.fillScreen(ST77XX_BLACK);
Serial.println(tft.getRotation(), DEC);
tft.drawRoundRect(20, 10, 25, 15, 5, ST77XX_BLUE);
delay(500);
tft.setRotation(tft.getRotation()+1);
}
}
void rotateFillRoundRect(void) {
for (uint8_t i=0; i<4; i++) {
tft.fillScreen(ST77XX_BLACK);
Serial.println(tft.getRotation(), DEC);
tft.fillRoundRect(20, 10, 25, 15, 5, ST77XX_CYAN);
delay(500);
tft.setRotation(tft.getRotation()+1);
}
}
void rotateChar(void) {
for (uint8_t i=0; i<4; i++) {
tft.fillScreen(ST77XX_BLACK);
Serial.println(tft.getRotation(), DEC);
tft.drawChar(25, 15, 'A', ST77XX_WHITE, ST77XX_WHITE, 1);
delay(500);
tft.setRotation(tft.getRotation()+1);
}
}
void rotateString(void) {
for (uint8_t i=0; i<4; i++) {
tft.fillScreen(ST77XX_BLACK);
Serial.println(tft.getRotation(), DEC);
tft.setCursor(8, 25);
tft.setTextSize(1);
tft.setTextColor(ST77XX_WHITE);
tft.print("Adafruit Industries");
delay(500);
tft.setRotation(tft.getRotation()+1);
}
}
Testcode “QRCODE” using adafruit library and qrcode generator #
Source: https://github.com/felixerdy/QRCodeGenerator
#include <Adafruit_GFX.h> // Core graphics library
#include <Adafruit_ST7735.h> // Hardware-specific library for ST7735
#include <SPI.h>
#include "QRCodeGenerator.h"
#define TFT_CS D3 // 21
#define TFT_RST D2 // Of -1 als je de reset pin niet gebruikt
#define TFT_DC D4
Adafruit_ST7735 tft = Adafruit_ST7735(TFT_CS, TFT_DC, TFT_RST);
void setup() {
Serial.begin(9600);
Serial.println("QR Code op Adafruit ST7735 scherm");
tft.initR(INITR_144GREENTAB); // Initialiseer het scherm
tft.setRotation(1); // Pas aan indien nodig
tft.fillScreen(ST77XX_WHITE);
// QR-code genereren en tonen
drawQRCode("https://domoticx.net");
}
void drawQRCode(const char* text) {
QRCode qrcode;
uint8_t qrData[qrcode_getBufferSize(3)]; // QR-code versie 3 (29x29 pixels)
qrcode_initText(&qrcode, qrData, 3, ECC_LOW, text);
int qrSize = qrcode.size;
int scale = 4; // Pas aan voor een grotere QR-code
int offsetX = (tft.width() - qrSize * scale) / 2;
int offsetY = (tft.height() - qrSize * scale) / 2;
for (int y = 0; y < qrSize; y++) {
for (int x = 0; x < qrSize; x++) {
tft.fillRect(offsetX + x * scale, offsetY + y * scale, scale, scale,
qrcode_getModule(&qrcode, x, y) ? ST77XX_BLACK : ST77XX_WHITE);
}
}
}
void loop() {
delay(50);
// Geen code nodig in loop
}
Result
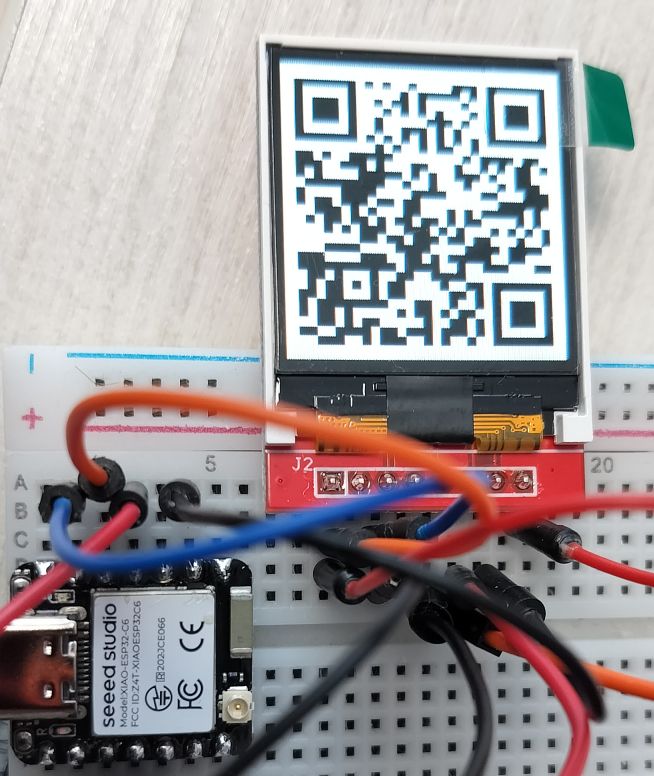
Products #
Sources #
None